LINQ, or Language Integrated Query, is a powerful feature of the .NET framework that allows developers to write queries in a more readable and concise manner. This blog post aims to delve into what LINQ is, its various advantages, and how it can be effectively used in .NET development.
What is LINQ?
LINQ, introduced with .NET Framework 3.5, stands for Language Integrated Query. It’s a set of extensions to the .NET Framework that encompasses language-integrated query, set, and transform operations. It can be used with any .NET language (like C# or VB.NET).
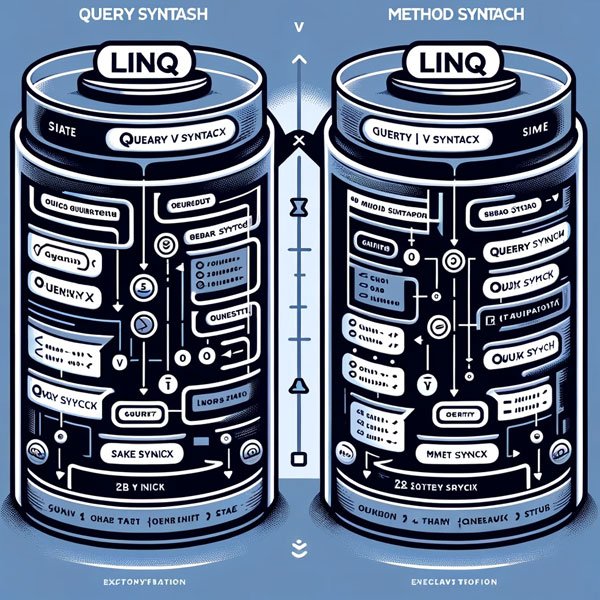
Key Features:
- Unified Query Syntax: LINQ provides a consistent querying experience across various data sources (such as SQL databases, XML documents, and in-memory collections).
- Compile-Time Safety: Queries are checked at compile-time, reducing runtime errors.
- Intellisense Support: LINQ integrates with Visual Studio’s Intellisense, providing autocomplete and syntax highlighting features.
Advantages of LINQ
1. Readability and Maintainability
LINQ queries are often more readable and concise than traditional query languages like SQL. This readability makes the code easier to understand and maintain.
2. Strong Typing
LINQ is strongly typed, which provides compile-time checking of queries and reduces runtime errors.
3. Interoperability Across Data Sources
With LINQ, the same query syntax can be used to query different data sources, such as SQL databases, XML documents, and in-memory collections like Lists or Arrays.
4. Debugging Support
LINQ integrates seamlessly with .NET’s debugging tools, allowing developers to step through queries and inspect results at runtime.
LINQ Query Syntaxes
LINQ supports two types of syntax:
- Query Syntax: Resembles SQL and is easy to read.
- Method Syntax (Fluent API): Uses extension methods and lambda expressions.
Example:
// Query Syntax
var querySyntax = from s in students
where s.Age > 18
select s.Name;
// Method Syntax
var methodSyntax = students.Where(s => s.Age > 18)
.Select(s => s.Name);
Both yield the same result but are different in style. Query syntax is generally more readable, especially for those familiar with SQL.
Real-World Application of LINQ
Data Filtering
LINQ is incredibly useful for filtering and extracting information from collections. For instance, fetching records from a database where a certain condition is met.
Aggregation
LINQ provides built-in methods for common aggregate functions like Count, Max, Min, Sum, and Average, simplifying data analysis tasks.
Joining Data
LINQ makes it easier to join data from multiple sources, something that’s extremely useful in complex applications.
LINQ is an indispensable part of the .NET framework, offering a range of benefits from improved readability to strong typing. Its ability to interact with various data sources using a unified syntax makes it a versatile tool for .NET developers.
Make sure to research more common .NET interview questions.